在JavaScript中,继承是一个核心概念,用于创建一个对象(称为子对象)可以继承另一个对象(称为父对象)的属性和方法的设计模式。尽管JavaScript不像传统的面向对象语言那样直接支持类和继承,但它提供了一些独特的机制来实现继承。以下是几种在JavaScript中实现继承的方法:
原型链继承
原型链继承是JavaScript中最常用的继承模式之一。它基于每个对象都有一个指向另一个对象的引用,这个引用被称为原型。
function Parent() {
this.parentProperty = true;
}
Parent.prototype.getParentProperty = function() {
return this.parentProperty;
};
function Child() {
this.childProperty = false;
}
// 继承Parent
Child.prototype = new Parent();
// 创建Child的一个实例
var childInstance = new Child();
console.log(childInstance.getParentProperty()); // 输出 true
注意: 原型链继承的缺点是如果父对象的属性是引用类型,那么一个子对象的修改会影响所有的子对象。
构造函数继承
为了解决原型链继承的缺点,可以使用构造函数继承。这种方法不使用原型,而是在子类构造函数中调用父类构造函数。
function Parent(name) {
this.name = name;
this.colors = ['red', 'blue', 'green'];
}
function Child(name) {
// 继承Parent,并传递参数
Parent.call(this, name);
}
var child1 = new Child('child1');
child1.colors.push('yellow');
console.log(child1.name); // 输出 'child1'
console.log(child1.colors); // 输出 ['red', 'blue', 'green', 'yellow']
注意: 构造函数继承的一个缺点是方法在每个实例上都是唯一的,因此对于每个子对象,父对象的方法都会被重新定义。
组合继承
组合继承是原型链继承和构造函数继承的组合,它利用了两种继承方式的优点。
function Parent(name) {
this.name = name;
this.colors = ['red', 'blue', 'green'];
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child(name, age) {
// 构造函数继承
Parent.call(this, name);
this.age = age;
}
// 原型链继承
Child.prototype = new Parent();
Child.prototype.constructor = Child;
Child.prototype.sayAge = function() {
console.log(this.age);
};
var child2 = new Child('child2', 18);
child2.colors.push('black');
child2.sayName(); // 输出 'child2'
child2.sayAge(); // 输出 18
原型式继承
原型式继承适用于那些不需要单独创建构造函数,但仍然需要在对象间共享信息的场景。
var parent = {
name: 'parent',
colors: ['red', 'blue', 'green'],
};
var child = Object.create(parent);
child.name = 'child';
console.log(child.name); // 输出 'child'
console.log(child.colors); // 输出 ['red', 'blue', 'green']
注意: 与原型链继承类似,这种方法也有相同的缺点,即引用类型的属性会在所有对象间共享。
寄生式继承
寄生式继承是对原型式继承的封装,添加了对象创建的封装过程。
function createAnother(original) {
var clone = Object.create(original);
clone.sayHi = function() {
console.log('hi');
};
return clone;
}
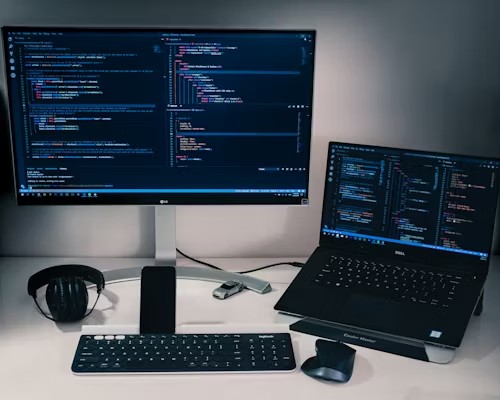
var person = {
name: 'person',
friends: ['Shelby', 'Court', 'Van']
};
var anotherPerson = createAnother(person);
anotherPerson.sayHi(); // 输出 'hi'
寄生组合式继承
寄生组合式继承是当前最理想的继承方法,它避免了组合继承中调用两次父构造函数的缺点。
function inheritPrototype(childObject, parentObject) {
var prototype = Object.create(parentObject.prototype);
prototype.constructor = childObject;
childObject.prototype = prototype;
}
function Parent(name) {
this.name = name;
this.colors = ['red', 'blue', 'green'];
}
Parent.prototype.sayName = function() {
console.log(this.name);
};
function Child(name, age) {
Parent.call(this, name);
this.age = age;
}
inheritPrototype(Child, Parent);
Child.prototype.sayAge = function() {
console.log(this.age);
};
var child3 = new Child('child3', 21);
child3.sayName(); // 输出 'child3'
child3.sayAge(); // 输出 21
这篇文章概述了JavaScript中实现继承的不同方法,并提供了每种方法的代码示例。每种方法都有其优点和缺点,选择哪一种取决于