Vue.js 是一种流行的前端框架,它专注于构建用户界面和单页应用程序。在 Vue.js 中,与后端服务进行通信通常是通过接口(API)来完成的。下面将详细介绍在 Vue 中如何使用接口。
1. 使用 `axios` 发起 HTTP 请求
`axios` 是一个基于 Promise 的 HTTP 客户端,用于浏览器和 node.js,它是一个非常流行的库,经常与 Vue.js 一起使用。
首先,你需要安装 `axios`:
npm install axios
然后,在你的 Vue 组件或服务中,你可以这样使用它:
// 引入 axios
import axios from 'axios';
// 创建 Vue 实例
export default {
name: 'App',
data() {
return {
info: null
};
},
created() {
this.fetchData();
},
methods: {
async fetchData() {
try {
// 使用 axios 发起 GET 请求
const response = await axios.get('https://api.example.com/data');
this.info = response.data;
} catch (error) {
console.error(error);
}
}
}
};
2. 使用 Vue Router 进行页面跳转
当你需要根据接口返回的结果进行页面跳转时,你可以利用 Vue Router:
// 引入 router
import router from './router';
// 接口调用后根据条件跳转
async fetchData() {
try {
const response = await axios.get('https://api.example.com/data');
if (response.data.needsRedirect) {
// 使用 router 进行跳转
router.push({ name: 'OtherPage' });
}
} catch (error) {
console.error(error);
}
}
3. 处理错误和加载状态
在实际应用中,你需要考虑接口请求的加载状态和可能出现的错误。
data() {
return {
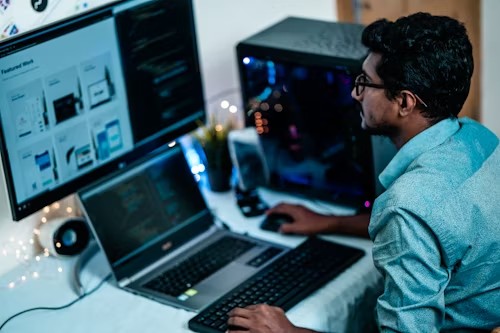
isLoading: false,
info: null,
error: null
};
},
methods: {
async fetchData() {
this.isLoading = true;
try {
const response = await axios.get('https://api.example.com/data');
this.info = response.data;
} catch (error) {
this.error = error;
} finally {
this.isLoading = false;
}
}
}
4. 使用接口进行表单提交
对于表单提交,你可以使用 `axios` 发起 POST 请求:
methods: {
async handleSubmit() {
try {
const response = await axios.post('https://api.example.com/data', this.form);
// 处理响应数据
} catch (error) {
// 处理错误
}
}
}
5. 接口封装
对于大型项目,建议对接口进行封装,以便于维护和重用。
// apiService.js
import axios from 'axios';
export const fetchData = async () => {
const response = await axios.get('https://api.example.com/data');
return response.data;
};
// 在组件中使用
import { fetchData } from './apiService';
async fetchData() {
try {
this.info = await fetchData();
} catch (error) {
console.error(error);
}
}
6. 注意事项
- 为了防止跨域请求问题,你可能在开发过程中需要配置代理(Proxy)。
- 对于安全性要求较高的接口,需要配置相应的认证措施,如 OAuth、JWT 等。
- 对于接口请求频繁的情况,考虑实现缓存机制。
通过上述介绍,你应该对在 Vue.js 中如何使用接口有了更深入的理解。记住,实际开发中需要根据项目具体需求进行适当的调整和优化。